AngularJS Services: AngularJS uses individualized objects called services to perform specialized tasks. Filters and controllers rely on services on an as-needed basis. Services typically use the dependency injection mechanism. There are many services built into AngularJS, such as $route, $location, $window, $http, etc., each in control of a different task.
The $http service, for example, contains everything necessary to access and collect data from a web server and pass it on to the $route service, which in turn defines it. You can also create custom services and reuse them throughout your code. Built-in services are easy to identify in code because they always begin with the dollar sign ($).
AngularJS Services Example
<!DOCTYPE html> <html> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js></script> <body> <div ng-controller="exampleCtrl" ng-app="exampleApp"> <p>The url address of this page is:</p> <h3>{{exampleUrl}}</h3> </div> <script> var app = angular.module('exampleApp', []); app.controller('example Ctrl', function($scope, $location) { $scope.example Url = $location.absUrl(); }); </script> </body> </html>
Angular JS Services Example Output
<p>The url address of this page is:</p>
https://tutorials.freshersnow.com/angular-js/angular-js-services-with-example/
The $http Service
The $http service is a commonly-used service in AngularJS. A request to the server is initiated by the service and the application handles the response.
The $http Service Example
Request data from the server using the $http service:
<!DOCTYPE html> <html> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <body> <div ng-app="example App" ng-controller="exampleCtrl"> <h1>{{exampleWelcome}}</h1> </div> <script> var app = angular.module('exampleApp', []); app.controller('exampleCtrl', function($scope, $http) { $http.get("welcome.htm").then(function (response) { $scope.exampleWelcome = response.data; }); }); </script> </body> </html>
The $http Service Example Output
Welcome to AngularJS!
The $timeout Service
Here, we use a window.setTimeout() function to get $timeout service.
$timeout Service Example
<!DOCTYPE html> <html> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script> <body> <div ng-app="myApp" ng-controller="myCtrl"> <p>This header will change after two seconds:</p> <h1>{{myHeader}}</h1> </div> <p>The $timeout service runs a function after a specified number of milliseconds.</p> <script> var app = angular.module('myApp', []); app.controller('myCtrl', function($scope, $timeout) { $scope.myHeader = "Hello!"; $timeout(function () { $scope.myHeader = "Welcome to tutorial.Freshersnow.com"; }, 2000); }); </script> </body> </html>
$timeout Service Example Output
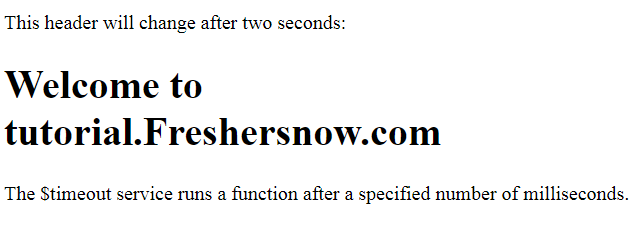
$interval Service
The window.setInterval function is used for $interval service.
$interval Service Example
<!DOCTYPE html> <html> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script> <body> <div ng-app="myApp" ng-controller="myCtrl"> <p>The time is:</p> <h1>{{theTime}}</h1> </div> <p>The $interval service runs a function every specified millisecond.</p> <script> var app = angular.module('myApp', []); app.controller('myCtrl', function($scope, $interval) { $scope.theTime = new Date().toLocaleTimeString(); $interval(function () { $scope.theTime = new Date().toLocaleTimeString(); }, 1000); }); </script> </body> </html>
$interval Service Example Output
Create Own Service
Connect your service to the module to create your own service.
Create Own Service Example
<!DOCTYPE html> <html> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script> <body> <div ng-app="myApp" ng-controller="myCtrl"> <p>The hexadecimal value of 10 is:</p> <h1>{{hex}}</h1> </div> <p>A custom service with a method that converts a given number into a hexadecimal number.</p> <script> var app = angular.module('myApp', []); app.service('hexafy', function() { this.myFunc = function (x) { return x.toString(16); } }); app.controller('myCtrl', function($scope, hexafy) { $scope.hex = hexafy.myFunc(10); }); </script> </body> </html>