AngularJS Forms: The forms in AngularJS are used for validation of input controls and help with data-binding and. When a form object is found by the AngularJS compiler, the compiler uses the ngForm directive to create a form controller.
The form controller object then searches for all input elements and creates the controls for each. What is required next is a data model attribute in order to establish two-way data binding, which will give the user instant feedback through the AngularJS validation methods.
AngularJS Forms Built-in Validation Methods
There are 14 built-in validation methods in AngularJS. These methods work well with HTML input elements and are dependable across all major web browsers.
Input Controls
The input controls are the HTML input elements:
- Input elements
- Select elements
- Button elements
- Textarea elements
Data-Binding-Input controls use the ng-model directive for data-binding.
AngularJS Form Example
<!DOCTYPE html> <html lang="en"> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <body> <div ng-app="example App" ng-controller="formCtrl"> <form novalidate> First_Name:<br> <input type="text" ng-model="user.firstName"><br> Last_ Name:<br> <input type="text" ng-model="user.last_name"> <br><br> <button ng-click "reset()"> RESET</button </form> <p>form = {{user}]</p> <p>master = {{master}}</p> </div> <script> var app = angular.module('exampleApp', []); app.controller('formCtrl', function($scope) { $scope.master = {firstName:"eBook",last_name:"Reader"}; $scope.reset = function() { $scope.user= angular.copy($scope.master); }; $scope.reset(); }); </script> </body> </html>
Angular JS Form Example Output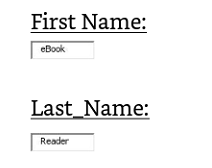
reset() Method Example
form = {"firstName":"eBook", "last_name":"Reader"} master = ["firstName":"eBook","last name":"Reader"}
Example Explanation
“Novalidate” disables default browser validation. The directive, ng-app, defines the application. The directive, ng-controller, defines the AngularJS application controller. The directive, ng-model, sync input elements in the model. The controller, formerly, defines the reset() method and gives values to the master object. The method, reset(), makes the user and master objects equal. The directive, ng-click, calls the reset() method if the button is clicked.