AngularJS Filters: Filters format data in the application. AngularJS includes several filters to transform data and that are listed below.
AngularJS Filters
- orderBy- Orders arrays.
- currency- Format numbers to currency.
- date- Formats dates.
- filter- Selects parts of an array.
- number- Format numbers to strings.
- JSON- Formats object to JSON strings.
- limit To- Limits strings or arrays, to specific numbers.
- lowercase- Format string to lowercase.
- uppercase- Format string to uppercase.
Adding Filters to Expressions
Filters are added by the “pipe” symbol | in front of the filter.
In this example, we will look at a filter for upper-case letters:
Adding AngularJS Filters to Expression Example
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script> </head> <body ng-app > <h1>AngularJS Uppercase/Lowercase Filter Demo: </h1> <div ng-init="person.firstName='Tutorials';person.lastName='.Freshersnow'"> Lower case: {{person.firstName + ' ' + person.lastName | lowercase}} <br /> Upper case: {{person.firstName + ' ' + person.lastName | uppercase}} </div> </body> </html>
Adding Filters to Expressions Example Output
Adding Filters to Directives
Directives accept Filters like ng-repeat with the help of pipe Symbol.
Adding Filters to Directives Example
<!DOCTYPE html <html> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"> </script> <body> <div ng-controller="nameCtrl"ng- app="exampleApp"> <p>Looping with objects:</p> <ul> <li ng-repeat="x in names | orderBy:'state'"> {{x.name + ','+ x.state }} </li> </ul> </div> <script> angular.module(example App',[]).controller('name Ctrl', function($scope) { $scope.names = [ {name:'Lisa',state: 'Illinois'}, {name:'Carl',state:'Maryland'}, {name:'Susan',state:'Texas'}, {name:'Lisa',state:'Georgia'}, {name:'Mick',state:'Massachusetts'}, {name:Gary,state: Florida'}, {name:'Marcia',state:'Louisiana'}, ]; }); </script> </body> </html>
Adding Filters to Directives Example Output
The “Filter” Filter
The “filter” filter is used to parse subsections of arrays.
The “Filter” Example
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.16/angular.min.js"></script> </head> <body ng-app="myApp" > <h1>AngularJS Filter Demo: </h1> <div ng-controller="myController"> Enter Name to search: <input type="text" ng-model="searchCriteria" /> <br /> Result: {{myArr | filter:searchCriteria}} </div> <script> var myApp = angular.module('myApp', []); myApp.controller("myController", function ($scope) { $scope.myArr = ['C', 'C++', 'Java', 'R Lang', 'Python', 'HTML'] }); </script> </body> </html>
The “Filter” Example Output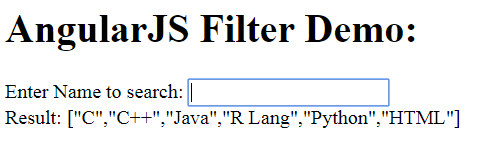
Custom Filters in AngularJS
By registering a function called a “filter factory” with your module, you can create your own custom filters: In this example, we will create a unique filter that will make every other letter uppercase.
Custom Filter Example
<!DOCTYPE html> <html> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <body> <ul ng-app="example App" ng-controller "nameCtrl"> <li ng-repeat="y in names"> {{y | example Filter}} </li> </ul> <script> var app angular.module(example App', []); app.filter(example Filter, function() { return function(y) { var i, c, txt= ""; for (i 0; i < y.length; i++) { c =x[i]; if (i % 2 == 0) { c = c.toUpperCase(); } txt += c; } return txt; }; }); app.controller('name app.controller('name Ctrl', function($scope) { $scope.names = [ 'Jan', 'Carl', 'Maggy', 'Heather', 'joe', 'Gary', 'Terrance', 'Marc', ]; </script> </html>