NodeJS Callback: A callback is a function in the NodeJS that will be executed when the task is completed and prevents blocking and allow other codes to run in meanwhile. The callback function is called after the task gets completed and it is an asynchronous correspondent for a function. The Callback concept will make the NodeJS scalable because it can handle more requests without waiting for any function to return the results.
For example, if a function starts reading a file, it returns control of the execution environment that helps to execute the next instruction. Once I/O operations are completed on file, the callback function will be called that avoids blocking or wait for file I/O operations.
NodeJS Callback Sample Examples
Let’s consider a file named sampleinput.txt and that contains the information like the following.
Hello Programmers!!! Learn NodeJS with Tutorial.freshersnow
Synchronous Example
Use the following code to create sync.js.
// Write JavaScript code var fs = require("fs"); var filedata = fs.readFileSync('sampleinput.txt'); console.log(filedata.toString()); console.log("End of Program execution");
Example Explanation
Here the “fs” library will handle file-system operations. The readFileSync() function blocks execution until the file gets completed and it is synchronous.
Synchronous Example Output
Asynchronous Example
Consider the same sample file named sampleinput.txt and the data in that is as follows.
Hello Programmers!!! Learn NodeJS with Tutorial.freshersnow
Use the following code to create async.js.
// Write a JavaScript code var fs = require("fs"); fs.readFile('sampleinput.txt', function (ferr, filedata) { if (ferr) return console.error(ferr); console.log(filedata.toString()); }); console.log("End of Program execution");
Example Explanation
The “fs” library is used to handle file-system operations. The readFile() function is asynchronous and returns the control immediately to the next instruction while the function runs in the background. The callback function will be transformed into the called task when the running background tasks are finished.
Asynchronous Example Output: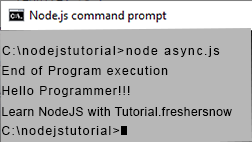
That’s how the callback function works synchronously and asynchronously in NodeJS.