NodeJS Buffers: What is a Buffer? A buffer is just the memory area. The JavaScript developers are not familiar with the buffers but atleast less than C, C++ and Go Language Developers. It provides a fixed size of memory that can be used outside of the V8 JavaScript engine. You may assume that buffer is like an array of integers that represent a byte of data. It can be implemented by the Node Buffer class.
NodeJS Buffer Uses
- At first, the buffers are introduced to be helpful for developers to access binary data in the ecosystem because the traditional method gets access to strings, not binaries.
- The buffers are linked with Streams. When the Stream processor receives data, it immediately transfers the data in a buffer.
- For example, a simple visual representation of buffer is while you watching any video on YouTube then the red line goes far away from the visual point. Similarly downloading data faster than you are viewing it.
Creating a Buffer in Node.js
The multiple ways of Node JS Buffer will help you to create a buffer. The three most commonly used methods are mentioned below.
Uninitiated Buffer
The following syntax is used for creating an uninitiated buffer for 15 octets.
var buf = new Buffer(15);
Buffer from String
The syntax specifies the buffer creation from string with encoding type (optional)
var buf = new Buffer("Tutorials.freshernow","utf=8");
Buffer from Array
The below syntax is used to create a buffer from the already provided array.
var buf = new Buffer([10,15,20,25,30]);
Writing to Buffers in NodeJS
To write data into the node buffer, the buf.write() method is used.
buf.write() Method Syntax
buf.write(String, offset, length, encoding)
buf.write() Parameters Explanation
- string: To define the string data that written into the buffer.
- offset: To provide the index where the buffer starts writing. The default value is 0.
- length: It defines the number of bytes to write the data. The Default value is buffer.lenth.
- Encoding: It provides the encoding mechanism and its default value is “utf-8”.
The buf.write() method is used in strings that will return the number of octets. In case the buffer doesn’t contain the required space for the string then it will write a part of the string.
buf.write() Example
// Write JavaScript code here cbuf = new Buffer(256); bufferlen = cbuf.write("Tutorials.freshernow"); console.log("No. of Octets in which string is written : "+ bufferlen);
buf.write() Example Output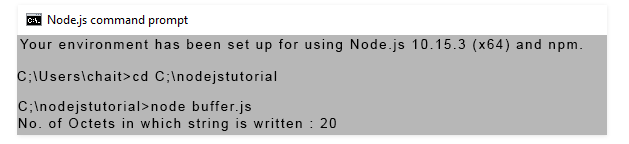
Reading from Buffers in NodeJS
To read the data from the buffer in Node JS use buf.toString() method.
buf.toString() Syntax
buf.toString(encoding, start, end)
buf.toString() Parameters Explanation
- encoding: It provides the encoding mechanism and its default value is “utf-8”.
- start: It provides the index to start reading and its default value is 0.
- end: It provides the index that to end reading and its default value is a complete buffer.
buf.toString() Example
// Write JavaScript code here rbuf = new Buffer(26); var j; for (var i = 65, j = 0; i < 90, j < 26; i++, j++) { rbuf[j] = i ; } console.log( rbuf.toString('utf-8',3,9));
buf.toString() Example Output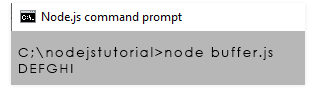
To copy a buffer
The NodeJS allows you to copy a buffer with the help of a copy() method.
copy() Method Example
const buf = Buffer.from('Hey!') let bufcopy = Buffer.alloc(4) //allocate 4 bytes buf.copy(bufcopy)
To copy from the particular starting point to endpoint then use three more parameters.
const buf = Buffer.from('Hey!') let bufcopy = Buffer.alloc(2) //allocate 2 bytes buf.copy(bufcopy, 0, 2, 2) bufcopy.toString() //'He'
To slice a buffer
The slicing of the buffer is creating partial visualization of a buffer. A slice is not copying the buffer, the original buffer will be the same as you created. The slice() method is used to create and the parameters are starting point and ending point(optional).
slice() Method Example
const buf = Buffer.from('Hey!') buf.slice(0).toString() //Hey! const slice = buf.slice(0, 2) console.log(slice.toString()) //He buf[1] = 111 //o console.log(slice.toString())
Length of Buffer
In Node JS, find the length of the buffer with the help of length property.
Length of Buffer Example
const buf = Buffer.from('Hey!') console.log(buf.length)
These are some operations that Node JS allows you to perform on Buffers.